Building Your First Generative AI Model: A Practical Guide
In today’s digital landscape, Generative AI has transformed from a research curiosity to a powerful tool driving innovation across industries. Whether you’re looking to create text, images, code, or other content, building your first generative AI model can seem daunting—but it doesn’t have to be.
This guide will walk you through the entire process of creating your own generative AI model, from understanding the fundamentals to deploying your creation. Let’s embark on this exciting journey together!
Understanding Generative AI: The Fundamentals
Before diving into code, let’s understand what makes generative AI tick. At its core, generative AI refers to algorithms that can create new content rather than simply analyzing existing data.

Unlike traditional AI models that classify, predict, or recognize patterns, generative models create something new. For beginners, I recommend starting with text generation models—they’re more accessible and require less computational resources than image or video generation.
Let’s break down how generative AI models learn:
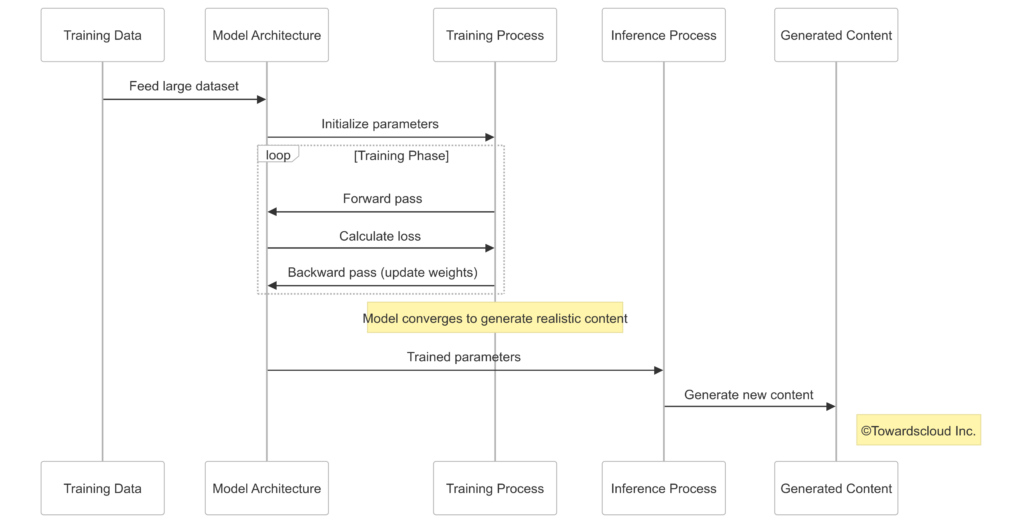
Choosing Your First Project: Text Generation with GPT-2
For your first generative AI project, I suggest building a text generation model using GPT-2. While newer models like GPT-4 exist, GPT-2 offers a perfect balance of capability and accessibility for beginners. It can run on consumer-grade hardware and still produce impressive results.
What You’ll Need:
- A computer with a decent GPU (though CPU-only is possible)
- Python programming knowledge (intermediate level)
- Familiarity with PyTorch or TensorFlow
- Dataset relevant to your domain of interest
- Patience (training takes time!)
Setting Up Your Environment
Let’s start by setting up a proper development environment. I’ll use Python with PyTorch and the Hugging Face Transformers library, which simplifies working with language models.
Setting Up Your Environment
# Create a virtual environment
python -m venv genai-env
# Activate the environment
# On Windows
genai-env\Scripts\activate
# On macOS/Linux
source genai-env/bin/activate
# Install required packages
pip install torch torchvision torchaudio
pip install transformers datasets
pip install matplotlib numpy tqdm
pip install tensorboard
pip install -U accelerate
pip install wandb # for experiment tracking (optional but recommended)
# Verify installations
python -c "import torch; print('PyTorch version:', torch.__version__); print('CUDA available:', torch.cuda.is_available())"
python -c "import transformers; print('Transformers version:', transformers.__version__)"
The commands above create an isolated Python environment and install all the necessary libraries. The last two commands verify that everything is working properly. If torch.cuda.is_available()
returns True
, you’re set to use your GPU for training.
Understanding the Process: From Data to Model
The journey from raw data to a working generative model follows several key steps. Let’s visualize this process:
Generative AI Development Pipeline
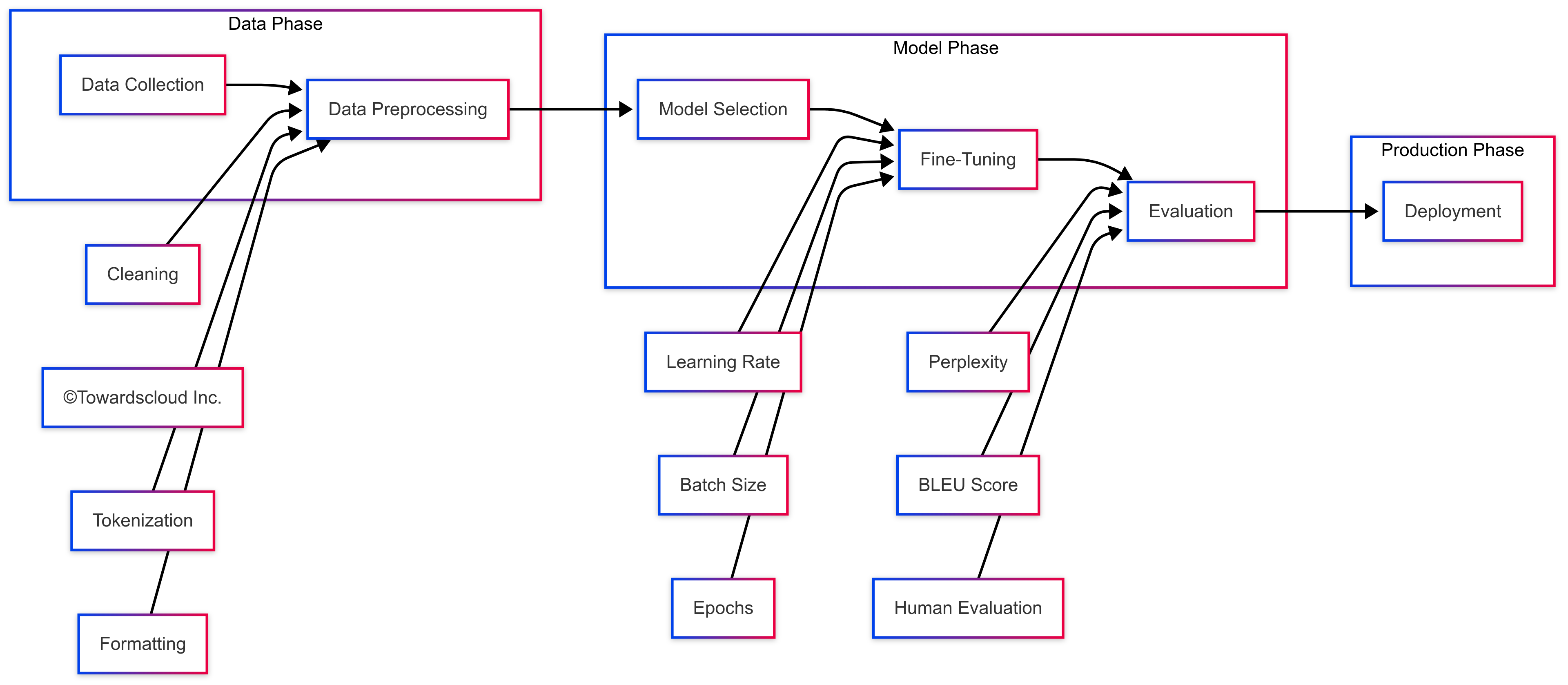
Data Collection and Preparation
Your generative model is only as good as the data it learns from. For text generation, you’ll need a substantial corpus of text in your domain of interest.
Let’s say we want to create a model that generates cloud computing documentation. We would need to collect relevant technical documentation, articles, and guides.
import os
import pandas as pd
from datasets import Dataset, DatasetDict
from transformers import GPT2Tokenizer
# Define paths
data_dir = "cloud_docs/"
output_dir = "processed_data/"
os.makedirs(output_dir, exist_ok=True)
# Load data (assuming we have text files in the data_dir)
def load_text_files(directory):
texts = []
for filename in os.listdir(directory):
if filename.endswith(".txt"):
file_path = os.path.join(directory, filename)
with open(file_path, 'r', encoding='utf-8') as f:
text = f.read()
texts.append({"text": text})
return texts
# Load and create a dataset
print("Loading data...")
train_texts = load_text_files(os.path.join(data_dir, "train"))
val_texts = load_text_files(os.path.join(data_dir, "val"))
train_dataset = Dataset.from_pandas(pd.DataFrame(train_texts))
val_dataset = Dataset.from_pandas(pd.DataFrame(val_texts))
dataset = DatasetDict({
"train": train_dataset,
"validation": val_dataset
})
print(f"Dataset created with {len(train_dataset)} training examples and {len(val_dataset)} validation examples")
# Initialize tokenizer
tokenizer = GPT2Tokenizer.from_pretrained("gpt2")
tokenizer.pad_token = tokenizer.eos_token # Define padding token
# Tokenization function
def tokenize_function(examples):
# Tokenize inputs
tokenized = tokenizer(
examples["text"],
padding="max_length",
truncation=True,
max_length=512,
return_tensors="pt"
)
return tokenized
# Apply tokenization to dataset
print("Tokenizing data...")
tokenized_datasets = dataset.map(
tokenize_function,
batched=True,
remove_columns=["text"]
)
# Save the processed data
tokenized_datasets.save_to_disk(output_dir)
print(f"Processed data saved to {output_dir}")
# Display a sample
print("\nSample tokenized input:")
sample = tokenized_datasets["train"][0]
print("Input IDs (first 10):", sample["input_ids"][:10])
print("Length of input:", len(sample["input_ids"]))
The code above performs several important tasks:
- It loads text files from designated directories.
- It creates a dataset structure with training and validation splits.
- It tokenizes the text using GPT-2’s tokenizer.
- It saves the processed data for later use.
Tokenization is particularly important in NLP—it’s the process of converting raw text into tokens (numbers) that the model can understand. The Hugging Face tokenizer handles this complexity for us.
Model Training: Fine-Tuning GPT-2
Rather than training a model from scratch (which would require enormous computational resources and data), we’ll use a technique called “fine-tuning.” This approach takes a pre-trained model and adapts it to our specific task and domain.
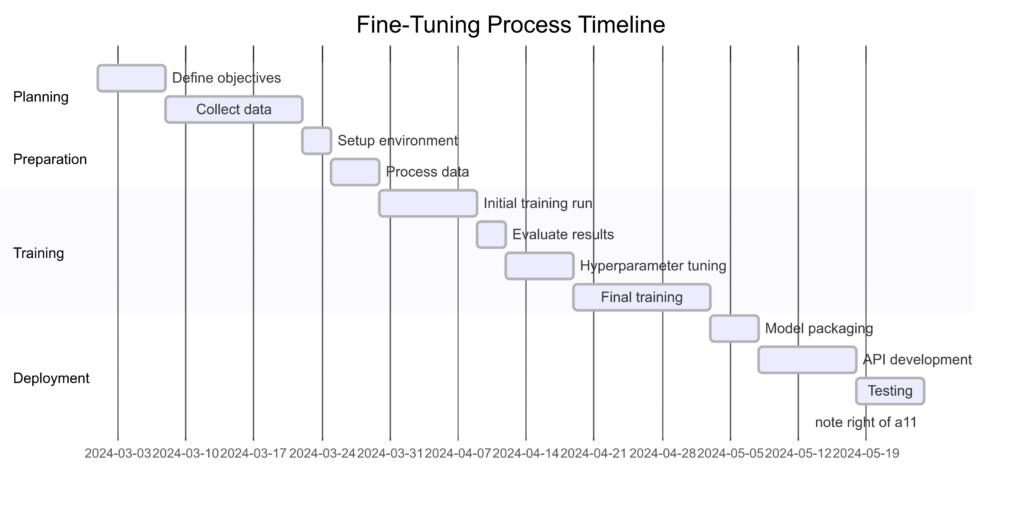
Now, let’s write the code to fine-tune GPT-2 on our prepared dataset:
Fine-Tuning GPT-2 Model
import os
import math
import torch
from datasets import load_from_disk
from transformers import (
GPT2LMHeadModel,
GPT2Tokenizer,
Trainer,
TrainingArguments,
DataCollatorForLanguageModeling
)
# Configuration
model_name = "gpt2" # You can use "gpt2-medium" for a larger model if you have more compute
data_dir = "processed_data/"
output_dir = "fine_tuned_model/"
os.makedirs(output_dir, exist_ok=True)
# Set up device
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
print(f"Using device: {device}")
# Load tokenizer and model
print("Loading tokenizer and model...")
tokenizer = GPT2Tokenizer.from_pretrained(model_name)
tokenizer.pad_token = tokenizer.eos_token # Define padding token
model = GPT2LMHeadModel.from_pretrained(model_name).to(device)
print(f"Model parameters: {model.num_parameters():,}")
# Load processed data
print("Loading data...")
tokenized_datasets = load_from_disk(data_dir)
# Create data collator for language modeling
data_collator = DataCollatorForLanguageModeling(
tokenizer=tokenizer,
mlm=False # GPT-2 uses causal language modeling (not masked)
)
# Define training arguments
training_args = TrainingArguments(
output_dir=output_dir,
overwrite_output_dir=True,
num_train_epochs=3, # Number of training epochs
per_device_train_batch_size=4, # Batch size for training
per_device_eval_batch_size=4, # Batch size for evaluation
eval_steps=500, # Number of steps between evaluations
save_steps=1000, # Number of steps between checkpoints
warmup_steps=500, # Number of warmup steps for learning rate scheduler
prediction_loss_only=True,
logging_dir="./logs", # Directory for storing logs
logging_steps=100,
learning_rate=5e-5, # Learning rate
weight_decay=0.01, # Weight decay for regularization
fp16=torch.cuda.is_available(), # Use mixed precision if a GPU is available
evaluation_strategy="steps", # Evaluate during training
save_total_limit=2, # Limit the total amount of checkpoints
report_to="tensorboard", # Report metrics to TensorBoard
)
# Create trainer
trainer = Trainer(
model=model,
args=training_args,
data_collator=data_collator,
train_dataset=tokenized_datasets["train"],
eval_dataset=tokenized_datasets["validation"],
)
# Train the model
print("Starting training...")
trainer.train()
# Save the model and tokenizer
print("Saving model...")
trainer.save_model(output_dir)
tokenizer.save_pretrained(output_dir)
# Calculate perplexity on validation set
print("Evaluating model...")
eval_results = trainer.evaluate()
print(f"Perplexity: {math.exp(eval_results['eval_loss']):.2f}")
print("Training completed!")
This script handles the entire fine-tuning process:
- It loads our pre-processed data from disk.
- It initializes a pre-trained GPT-2 model.
- It configures the training parameters—learning rate, batch size, number of epochs, etc.
- It trains the model on our custom dataset.
- It saves the fine-tuned model for later use.
- It evaluates the model’s performance using perplexity (a standard metric for language models).
Fine-tuning can take anywhere from hours to days, depending on your hardware and the size of your dataset.
Model Evaluation and Testing
After training, you’ll want to evaluate your model to see how well it performs. Perplexity is an important metric, but the true test is generating text and assessing its quality.
import torch
from transformers import GPT2LMHeadModel, GPT2Tokenizer
Load the fine-tuned model and tokenizer
model_dir = “fine_tuned_model/”
tokenizer = GPT2Tokenizer.from_pretrained(model_dir)
model = GPT2LMHeadModel.from_pretrained(model_dir)
Move model to GPU if available
device = torch.device(“cuda” if torch.cuda.is_available() else “cpu”)
model.to(device)
model.eval() # Set model to evaluation mode
Define generation function
def generate_text(prompt, max_length=200, num_return_sequences=3, temperature=0.7):
“””
Generate text based on a prompt.
Args:
prompt (str): The input prompt for text generation
max_length (int): Maximum length of the generated text
num_return_sequences (int): Number of different sequences to generate
temperature (float): Controls randomness. Lower means more deterministic.
Returns:
list: List of generated text sequences
"""
# Encode the prompt
input_ids = tokenizer.encode(prompt, return_tensors="pt").to(device)
# Generate text
output_sequences = model.generate(
input_ids=input_ids,
max_length=max_length,
temperature=temperature,
top_k=50,
top_p=0.95,
do_sample=True,
num_return_sequences=num_return_sequences,
pad_token_id=tokenizer.eos_token_id,
)
# Decode and return generated sequences
generated_texts = []
for sequence in output_sequences:
text = tokenizer.decode(sequence, skip_special_tokens=True)
generated_texts.append(text)
return generated_texts
Test with various prompts
test_prompts = [
“Cloud computing offers several benefits including”,
“The key differences between AWS and Azure are”,
“When implementing a serverless architecture, you should consider”,
]
Generate and print text for each prompt
for prompt in test_prompts:
print(f”\nPrompt: {prompt}”)
print(“-” * 50)
generated_texts = generate_text(prompt)
for i, text in enumerate(generated_texts):
print(f"\nGeneration {i+1}:")
print(text)
print("=" * 80)
Interactive mode
print(“\nInteractive Mode:”)
print(“Type ‘exit’ to quit.”)
while True:
user_prompt = input(“\nEnter a prompt: “)
if user_prompt.lower() == ‘exit’:
break
import torch
from transformers import GPT2LMHeadModel, GPT2Tokenizer
# Load the fine-tuned model and tokenizer
model_dir = "fine_tuned_model/"
tokenizer = GPT2Tokenizer.from_pretrained(model_dir)
model = GPT2LMHeadModel.from_pretrained(model_dir)
# Move model to GPU if available
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
model.to(device)
model.eval() # Set model to evaluation mode
# Define generation function
def generate_text(prompt, max_length=200, num_return_sequences=3, temperature=0.7):
"""
Generate text based on a prompt.
Args:
prompt (str): The input prompt for text generation
max_length (int): Maximum length of the generated text
num_return_sequences (int): Number of different sequences to generate
temperature (float): Controls randomness. Lower means more deterministic.
Returns:
list: List of generated text sequences
"""
# Encode the prompt
input_ids = tokenizer.encode(prompt, return_tensors="pt").to(device)
# Generate text
output_sequences = model.generate(
input_ids=input_ids,
max_length=max_length,
temperature=temperature,
top_k=50,
top_p=0.95,
do_sample=True,
num_return_sequences=num_return_sequences,
pad_token_id=tokenizer.eos_token_id,
)
# Decode and return generated sequences
generated_texts = []
for sequence in output_sequences:
text = tokenizer.decode(sequence, skip_special_tokens=True)
generated_texts.append(text)
return generated_texts
# Test with various prompts
test_prompts = [
"Cloud computing offers several benefits including",
"The key differences between AWS and Azure are",
"When implementing a serverless architecture, you should consider",
]
# Generate and print text for each prompt
for prompt in test_prompts:
print(f"\nPrompt: {prompt}")
print("-" * 50)
generated_texts = generate_text(prompt)
for i, text in enumerate(generated_texts):
print(f"\nGeneration {i+1}:")
print(text)
print("=" * 80)
# Interactive mode
print("\nInteractive Mode:")
print("Type 'exit' to quit.")
while True:
user_prompt = input("\nEnter a prompt: ")
if user_prompt.lower() == 'exit':
break
generated_texts = generate_text(user_prompt, num_return_sequences=1)
print("\nGenerated Text:")
print(generated_texts[0])
This evaluation script:
- Loads your fine-tuned model.
- Defines a function to generate text based on input prompts.
- Tests the model with predefined prompts relevant to cloud computing.
- Provides an interactive mode where you can input your own prompts.
Let’s understand the key parameters that control text generation:
- temperature: Controls randomness. Lower values (e.g., 0.2) make the output more deterministic, while higher values (e.g., 1.0) introduce more variety.
- top_k and top_p: Control which tokens the model considers at each step. These help balance between creativity and coherence.
- max_length: Determines how long the generated text can be.
- num_return_sequences: How many different outputs to generate for each prompt.
Deploying Your Model as a Web Service
Now that you have a working model, let’s make it accessible via a simple API. We’ll use Flask to create a lightweight web service:
Deploying Model as a Web Service
from flask import Flask, request, jsonify
import torch
from transformers import GPT2LMHeadModel, GPT2Tokenizer
import time
import logging
# Configure logging
logging.basicConfig(
level=logging.INFO,
format='%(asctime)s - %(name)s - %(levelname)s - %(message)s',
handlers=[logging.FileHandler("api.log"), logging.StreamHandler()]
)
logger = logging.getLogger(__name__)
app = Flask(__name__)
# Load model and tokenizer
model_dir = "fine_tuned_model/"
logger.info(f"Loading model from {model_dir}")
try:
tokenizer = GPT2Tokenizer.from_pretrained(model_dir)
model = GPT2LMHeadModel.from_pretrained(model_dir)
# Move model to GPU if available
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
model.to(device)
model.eval() # Set model to evaluation mode
logger.info(f"Model loaded successfully. Using device: {device}")
except Exception as e:
logger.error(f"Error loading model: {str(e)}")
raise
@app.route('/health', methods=['GET'])
def health_check():
"""Simple health check endpoint"""
return jsonify({"status": "healthy", "model": "GPT-2 fine-tuned"})
@app.route('/generate', methods=['POST'])
def generate_text():
"""Generate text based on input prompt"""
start_time = time.time()
# Get request data
data = request.json
prompt = data.get("prompt", "")
max_length = int(data.get("max_length", 200))
temperature = float(data.get("temperature", 0.7))
num_sequences = int(data.get("num_sequences", 1))
logger.info(f"Received generate request. Prompt: '{prompt[:50]}...'")
# Validate input
if not prompt:
return jsonify({"error": "Prompt cannot be empty"}), 400
if max_length > 1000:
return jsonify({"error": "max_length cannot exceed 1000"}), 400
try:
# Encode the prompt
input_ids = tokenizer.encode(prompt, return_tensors="pt").to(device)
# Generate text
output_sequences = model.generate(
input_ids=input_ids,
max_length=max_length,
temperature=temperature,
top_k=50,
top_p=0.95,
do_sample=True,
num_return_sequences=num_sequences,
pad_token_id=tokenizer.eos_token_id,
)
# Decode generated sequences
generated_texts = []
for sequence in output_sequences:
text = tokenizer.decode(sequence, skip_special_tokens=True)
generated_texts.append(text)
# Log performance
elapsed_time = time.time() - start_time
logger.info(f"Generated {num_sequences} sequences in {elapsed_time:.2f}s")
return jsonify({
"generated_texts": generated_texts,
"prompt": prompt,
"execution_time_seconds": elapsed_time
})
except Exception as e:
logger.error(f"Error generating text: {str(e)}")
return jsonify({"error": str(e)}), 500
if __name__ == '__main__':
app.run(host='0.0.0.0', port=5000, debug=False)
This deployment script:
- Creates a Flask web application with two endpoints:
/health
for checking if the service is running/generate
for generating text based on input prompts
- Loads your fine-tuned model when the application starts
- Handles incoming requests, validates parameters, and returns generated text
- Includes logging and error handling for production reliability
To deploy this service, you’d run:
Running the API Service
# Install required packages
pip install flask gunicorn
# Run with Flask (development)
python app.py
# Or run with Gunicorn (production)
gunicorn -w 1 -b 0.0.0.0:5000 app:app
For a production deployment, you would want to:
- Containerize your application using Docker
- Deploy to a cloud service like AWS, GCP, or Azure
- Set up monitoring and auto-scaling
- Configure a proper domain and HTTPS
Let’s visualize the deployment architecture:
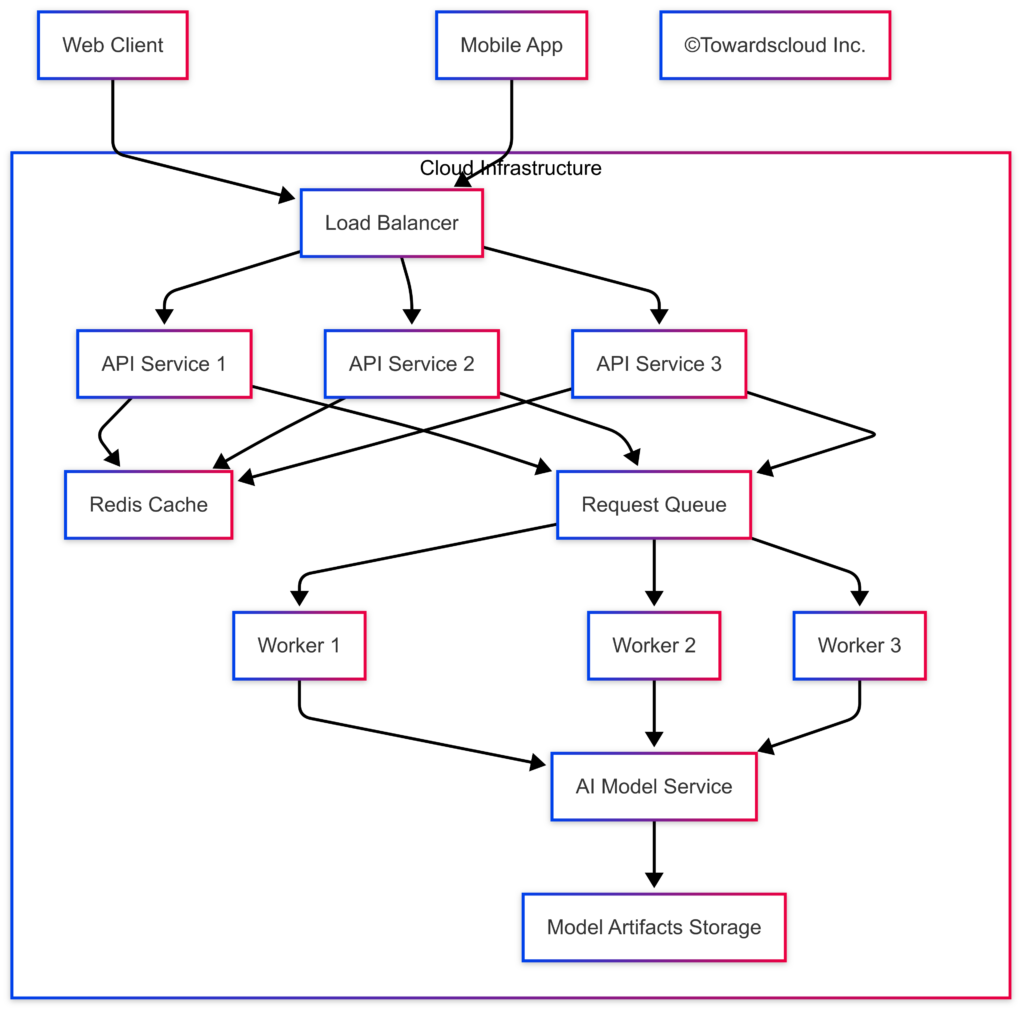
Going Beyond GPT-2: Advanced Techniques
As you grow more comfortable with building generative AI models, you might want to explore more advanced techniques:
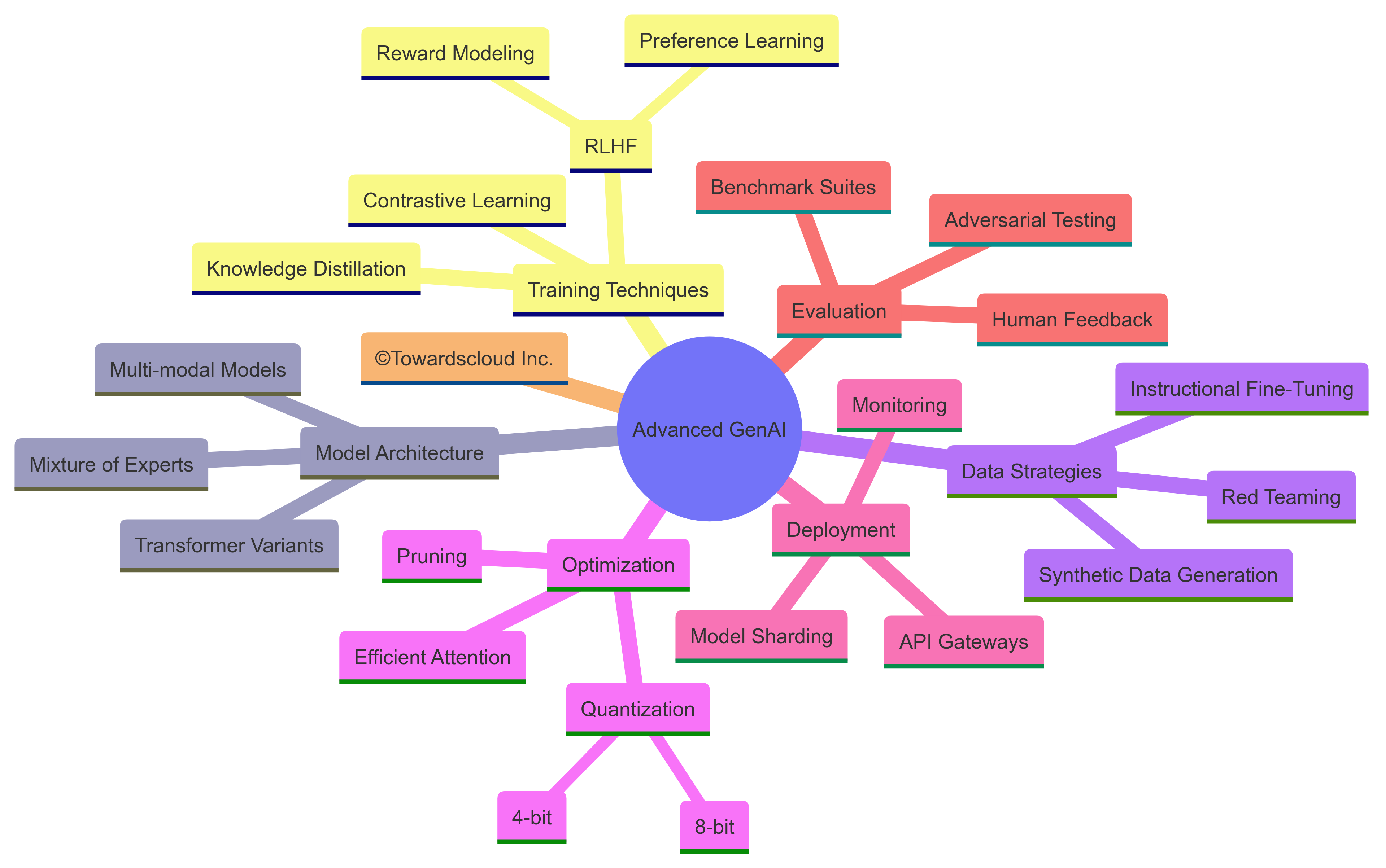
Practical Tips for Better Results
Based on my experience building generative models, here are some practical tips:
- Start small, then scale up: Begin with smaller models (like GPT-2) before attempting larger ones.
- Focus on data quality: Clean, diverse, high-quality data matters more than model size for specialized domains.
- Monitor training closely: Use TensorBoard or Weights & Biases to track loss curves and catch issues early.
- Use proper evaluation: Perplexity alone isn’t enough; have domain experts review your model’s outputs.
- Manage expectations: Even with fine-tuning, smaller models won’t match GPT-4’s capabilities. Understand your model’s limitations.
- Optimize for your hardware: Use mixed precision training (fp16) and gradient accumulation if you have limited GPU memory.
- Consider ethical implications: Ensure your model doesn’t generate harmful or biased content.
Real-World Example: Building a Technical Documentation Generator
Let’s tie everything together with a concrete example. Say you work for a cloud company and want to create a model that helps generate technical documentation.
Your process might look like this:
- Collect existing documentation from your company’s knowledge base
- Clean and preprocess the data, ensuring quality and consistency
- Fine-tune a GPT-2 model on this specialized data
- Create a simple web interface where technical writers can input partial documentation and get suggestions
- Implement feedback mechanisms so the model improves over time
This approach combines everything we’ve covered—data preparation, model training, deployment, and optimization—in a practical business context.
Conclusion
Building your first generative AI model is an exciting journey that combines technical knowledge with creativity. While it requires some programming skills and computational resources, the process has become increasingly accessible thanks to libraries like Hugging Face Transformers.
Remember that your first model won’t be perfect—and that’s okay! Each iteration is a learning opportunity. As you gain experience, you’ll develop an intuition for what works and what doesn’t, allowing you to build increasingly sophisticated models.
The field of generative AI is evolving rapidly, with new techniques and architectures emerging constantly. Stay curious, keep experimenting, and don’t hesitate to share your creations with the community.
What will you build with your generative AI model? The possibilities are limited only by your imagination.